Scriptcs gets new REPL commands and is now better than ever
Yesterday the scriptcs project got an little injection of awesomeness through a set of new REPL commands we have added. You can now conveniently access a bunch of contextual information about your REPL session - the idea is to make your work with the REPL smoother, more productive and, of course, more enjoyable. Let’s quickly walk through them in this post.
By the way, it’s a good moment to remind that the REPL command infrastructure is fully extensible - so you can easily build up your own commands. Watch my talk from NDC Oslo if you are interested, there is an example in there.
Razor views pre-compilation with ASP.NET 5 and MVC 6
In previous versions of MVC framework, running on top of the “classic” ASP.NET runtime, it was quite common for developers to switch view compilation on, so that the views get compiled upfront, allowing you to see any errors at compile time, rather than at runtime.
This was done by a simply adding
Given that everything changes in the new ASP.NET 5 world, how would you do it now? Let’s explore.
Formatters in ASP.NET MVC 6
One of the key concepts in HTTP API development is the notion of content negotiation (conneg). ASP.NET Web API provided first class support for content negotiation through the use of MediaTypeFormatters.
While MVC 6 is a de facto brand new framework, rebuilt from scratch, the majority of concepts from MVC 5 and Web API 2 have naturally been brought forward, and conneg done through formatters are one of them.
Let’s have a look at formatters in MVC6.
Open Source .NET – why should you care?
Moments ago, at VS Connect event, Microsoft, through the Corporate Vice President of the Developer Division S. Somasegar, dropped a bomb on the developer community by announcing that the .NET Core Framework, a new true cross platform .NET, has been open sourced (note, it’s still partial, not everything is there yet), and released under a very permissive MIT license. You can read up more on the .NET Core at the official blog.
This is a dramatic shift in an ecosystem that has traditionally been (fair or not) characterized as closed, often unfriendly to developers, Windows-specific (Mono is not, after all, a Microsoft project) and characterized by relatively high entry barriers (Visual Studio, lockdown to Windows Server etc).
I have a bunch of quick thoughts around this that I’d like to share.
Using ConfigR as a Configuration Source in ASP.NET vNext
One of the cool things about ASP.NET vNext is that it introudces a configuration abstraction, Microsoft.Framework.ConfigurationModel over your application’s configuration.
This is going to replace the old school, limiting approach of forcing you to work with XML configuration only through the System.Configuration classes.
Louis DeJardin has blogged about the ideas behind the isolation of Configuration in his blog post. In the meantime, let’s have a look at how we could enable a ConfigR-based configuration too.
Building refactoring tools (diagnostics and code fixes) with Roslyn
Some time ago I blogged about building next generation Visual Studio support tools with Roslyn. This was when Roslyn was still on its 2012 CTP. A lot has changed since then, with Roslyn going open source, and new iterations of the CTPs getting released.
Most of the APIs used in the original have changed, so I thought it would be a good idea to do a new post, and rebuilt the sample used in the old post from scratch, using the latest Roslyn CTP.
Route matching and overriding 404 in ASP.NET Web API
In ASP.NET Web API, if the incoming does not match any route, the framework is simply hard wired to return 404 to the client (or possibly pass through to the next configured middleware, in case of an OWIN hosted Web API). This is done immediately, without entering anywhere further down the pipeline (i.e. message handlers would not be invoked).
However, an interesting question was posted recently at StackOverflow - what if you want to override that hard 404, and given your specific routing requirements, respond to the client with a different status code if a specific route condition fails?
I already answered at StackOverflow, but decided this deserves a blog post regardless.
Over 100 ASP.NET Web API samples
As you might now by now, last month my ASP.NET Web API 2 Recipes book was released by Apress. The book contains over 100 recipes covering various Web API scenarios that aim to help you save some headaches when working on your current or next Web API project.
Each of the recipes has got an accompanying Visual Studio solution, which illustrates the given problem and presents a solution in a simple, isolated manner.
Obviously, it would be great if you went ahead and bought a book (then you would get an in-depth analysis of each case), but the source code itself is available for free at Github and as a download from Apress.
I hope it becomes a useful collection, illustrating how to deal with various Web API problems/scenarios.
Please note that some of the early examples might appear simple or even trivial - that’s the case with introductory recipes, where the gist of the matter is discussed in the book itself.
I have seen in some StackOverflow questions that the examples on Github have already helped a couple of folks, which is fantastic. You can find a summary of the samples below - they are structured in the book and in the repository the same way.
Things you didn’t know about action return types in ASP.NET Web API
When you are developing an ASP.NET Web API application, you can chose whether you want to return a POCO from your action, which can be any type that will then be serialized, an instance of HttpResponseMessage, or, since Web API 2, an instance of IHttpActionResult.
Let’s have a look at what really happens under the hood afterwards, and discuss some of the things about the response pipeline that you might have not known before.
Strongly typed direct routing link generation in ASP.NET Web API with Drum
ASP.NET Web API provides an IUrlHelper interface and the corresponding UrlHelper class as a general, built-in mechanism you can use to generate links to your Web API routes. In fact, it’s similar in ASP.NET MVC, so this pattern has been familiar to most devs for a while.
The main problem of it is that it’s based on magic strings, as, to generate a link, the route name has to be passed as a string literal. Moreover, all the parameters that are required to built up the link, are simply a set of name-values, represented by a dictionary or an anonymous object, which is hardly optimal. Code is not coherent, refactoring becomes a pain and in general error potential is high.
My friend, and one of the most respected folks in the Web API community, Pedro Felix, has created a library called Drum, designed to avoid the pitfalls of the UrlHelper, allowing you to build links for Web API direct routing (introudced in Web API 2) in a strongly typed way.
Drum works with any direct routing provider, including my own Strathweb.TypedRouting.
About
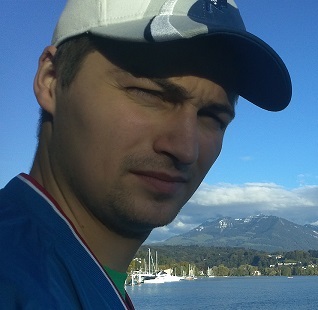
Hi! I'm Filip W., a software architect from Zürich 🇨🇭. I like Toronto Maple Leafs 🇨🇦, Rancid and quantum computing. Oh, and I love the Lowlands 🏴.
You can find me on Github, on Mastodon and on Bluesky.
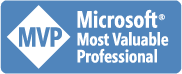
Recent Posts
- 2025/06/27, AI Agents with OpenAPI Tools - Part 2: Azure AI Foundry
- 2025/06/23, AI Agents with OpenAPI Tools - Part 1: Semantic Kernel
- 2025/06/06, A Cat Jumped on a Keyboard and Fixed Q# Array Syntax
- 2025/05/28, Exploring Microsoft Foundry Local
- 2025/04/25, Using Phi Silica in Windows App SDK on a Copilot Plus PC
Categories
- ai (25)
- ai agents (2)
- ai search (5)
- apache cordova (1)
- asp.net 5 (17)
- asp.net core (47)
- asp.net mvc (35)
- asp.net mvc 6 (7)
- asp.net vnext (6)
- asp.net web api (96)
- astronomy (1)
- autogen (1)
- azure (24)
- azure service bus (1)
- azure-devops (1)
- benchmark dotnet (1)
- bing maps (1)
- blazor (2)
- c plus (2)
- c-sharp (156)
- cryptography (6)
- csharp (6)
- csharp 10 (2)
- dnx (3)
- dotnet-cli (2)
- dotnet-script (11)
- duende (4)
- editorconfig (1)
- entity framework (2)
- espn api (2)
- events (1)
- ffi (4)
- fsharp (1)
- git (1)
- glimpse (1)
- html5 (4)
- identity server (2)
- iis (2)
- il (1)
- intro to qc (19)
- ios (7)
- javascript (9)
- jquery (4)
- jquery mobile metro (1)
- katana (2)
- kindle (1)
- knockout.js (8)
- kotlin (2)
- last.fm api (2)
- linq (1)
- mac (4)
- macos (1)
- mathematica (1)
- msbuild (3)
- mvc core (3)
- nancy (2)
- native (1)
- net (144)
- net 5 (3)
- net 6 (5)
- net 7 (7)
- net 8 (3)
- net 9 (1)
- net core (49)
- net sdk (2)
- ninject (2)
- odata (4)
- oidc (2)
- omnisharp (13)
- openai (12)
- osx (2)
- owin (5)
- phi (9)
- php (1)
- promptflow (1)
- python (1)
- q-sharp (38)
- qir (3)
- qiskit (1)
- quantum computing (43)
- roslyn (30)
- rust (6)
- scriptcs (11)
- scripting (9)
- security (9)
- semantic-kernel (1)
- servicestack (2)
- signalr (8)
- swift (9)
- testing (5)
- twitter boostrap (1)
- typescript (1)
- visual studio (4)
- visual studio code (11)
- wasi (3)
- wasm (3)
- windows (2)
- windows phone 7 (1)
- wordpress (1)
- wpf (2)