BSON (Binary JSON) and how your Web API can be even faster
I have been reading the wishlist at Web API Codeplex repository recently, and noticed that one of the most popular requested features, is to add support for BSON (Binary JSON) media type (6th on the [list][1]).
Of course all it takes to include BSON into your Web API is to simply write a media type formatter for it, and since JSON.NET already has great BSON support, it is actually quite easy.
Now, you might be asking a question, why to do it in the first place? Isn’t JSON enough? Well, the main reason is performance, as according to JSON.NET tests, BSON would produce output that’s often smaller than JSON (up to 25%). It is also much quicker to encode and decode, as for simple types there is no parsing to/from their string representation.
Let’s do it then.
Everything you want to know about ASP.NET Web API content negotiation
One of the key concepts in ASP.NET Web API, lying pretty much at the heart of it, is content negotiation - or simply conneg. I really believe that, before you start developing Web API solutions, you need to understand conneg well.
I thought it would be interesting to try to explain content negotiation in detail - what it is, what it does, and why it does that, especially as I have seen a lot of questions, misconceptions and misunderstandings around it on various boards or question sites.
Deploy your ASP.NET Web API application to Windows Azure in 3 minutes
Over the past months I have been blogging about ASP.NET Web API a lot. One question that I haven’t really addressed, is how to send this beast to production - because it’s one thing to develop something, and completely different to have it up and running in live environment.
While so many members of the community have really enjoyed Windows Azure since it has been unveiled in the new shape recently, a lot of people are still uncertain how to work with it - because, well, “cloud” has always sounded a little enterprise-like. I thought it might help people to have a quick step-by-step guide on how you could really easily deploy your app to Azure (using Git!).
More after the jump.
Run your favorite unit testing GUI directly from Visual Studio
If you are working a lot with unit tests and somehow are allergic to command line testing (I, for one, am) there is an easy way to configure your test project’s build to start your favorite’s library GUI automatically and load the test assembly into it.
This is very convenient and, as a bonus, allows you to set breakpoints in the test code, without having to attach to any processes.
Let’s have a quick look at how you can do that for xUnit and nUnit.
Using NLog to provide custom tracing for your ASP.NET Web API
One of the things I love most about MVC3, and now ASP.NET Web API, is that pretty much any functionality or service that is used by your application, can be replaced with a custom one. One of these is the entire tracing mechanism that Web API uses.
Let’s have a look today at how you can build a simple System.Web.Http.Tracing.ITraceWriter implementation to provide support for a custom logging framework in your Web API - in our case it will be NLog.
More after the jump.
Using controllers from an external assembly in ASP.NET Web API
In general, in my day-to-day job, I am working with large enterprise web applications, where clean projects, noise reduction and test driven development are holy. Therefore I have always been a big fan of placing MVC controllers outside the actual web application project, in a separate class library (or libraries).
With MVC it was very simple, but recently I faced this challenge in Web API, and turns out neither passing the assembly name through MapHttpRoute (not supported) or using something like ControllerBuilder.Current.DefaultNamespaces is not a viable option. So I turned to Henrik Nielsen for advice, and sure enough, he helped immediately. Let’s explore the solution.
Drag and drop files to WPF application and asynchronously upload to ASP.NET Web API
A while ago, I wrote a post on uploading files to ASP.NET Web API by dragging and dropping them to the browser. Today I will continue with the same topic, but instead, let’s build a WPF application to which you can drag and drop files, and they’ll get uploaded to ASP.NET Web API.
The important thing, is that we will use the *exact* same Web API upload controller as before, meaning the same controller will be handling uploads from the browser and from the client (WPF) application. The client will use the new HttpClient and it’s client-side DelegatingHandlers.
ASP.NET Web API integration testing with in-memory hosting
In-memory hosting is one of the hidden gems of ASP.NET Web API. While the community, forums, bloggers have been buzzing about web-host and self-host capabilities of Web API, aside from the terrific post by Pedro Felix, very little has been said about in memory hosting.
Let me show you an example today, how a lightweight Web API server can be temporarily established in memory (without elevated priviliges, or cannibalizing ports like self host) and used to perform integration testing, allowing you to test almost the entire pipeline, from the request to the response.
Control the execution order of your filters in ASP.NET Web API
One of the few minor annoyances in ASP.NET Web API is that you lack the ability to control the order in which the attributes/filters are executed. If you have several of those applied to one action, they will not run in the order they have been declared in the code, and that sometimes may cause a lot of problems.
In fact, as a member of the ASP.NET Web API Advisory Group I already brought that up during our meetings, not to mention it has already been submitted as an issue to the ASP.NET team on Codeplex. In the meantime, let’s tackle this problem and see how you can easily regain control over the execution order of the attributes.
Extending your ASP.NET Web API responses with useful metadata
If you ever worked with any API, which, in this day of age, you must have, you surely noticed that in most situations the API response isn’t just the result (requested data), but also a set of helpful metadata, like “total Results”, “timestamp”, “status” and so on.
In Web API, by default, you just serialize your models (or DTO) and such information are not present. Let’s build something which will solve this problem and help you decorate your response with hepful information. This would make it very easy for the client to implement paging, auto-loading scenarios, caching (if you return last modified information) and a lot more.
About
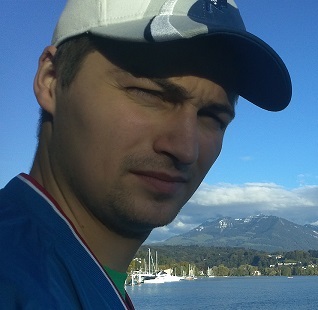
Hi! I'm Filip W., a cloud architect from Zürich 🇨🇭. I like Toronto Maple Leafs 🇨🇦, Rancid and quantum computing. Oh, and I love the Lowlands 🏴.
You can find me on Github and on Mastodon.
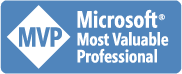
Recent Posts
- 2024/07/25, Announcing Strathweb Phi Engine - a cross-platform library for running Phi-3 anywhere
- 2024/07/12, Built-in support for Server Sent Events in .NET 9
- 2024/06/25, Announcing Q# Bridge - a library bringing the Q# simulator and tools to C#, Swfit and Kotlin
- 2024/05/09, Running Microsoft's Phi-3 Model in an iOS app with Rust
- 2024/04/19, Tool Calling with Azure OpenAI - Part 2: Using the tools directly via the SDK
Categories
- ai (11)
- ai search (4)
- apache cordova (1)
- asp.net 5 (17)
- asp.net core (45)
- asp.net mvc (35)
- asp.net mvc 6 (7)
- asp.net vnext (6)
- asp.net web api (96)
- astronomy (1)
- azure (19)
- azure service bus (1)
- azure-devops (1)
- benchmark dotnet (1)
- bing maps (1)
- blazor (1)
- c plus (2)
- c-sharp (154)
- cryptography (3)
- csharp (4)
- csharp 10 (2)
- dnx (3)
- dotnet-cli (2)
- dotnet-script (11)
- duende (2)
- editorconfig (1)
- entity framework (2)
- espn api (2)
- events (1)
- ffi (4)
- fsharp (1)
- git (1)
- glimpse (1)
- html5 (4)
- identity server (2)
- iis (2)
- il (1)
- intro to qc (19)
- ios (4)
- javascript (9)
- jquery (4)
- jquery mobile metro (1)
- katana (2)
- kindle (1)
- knockout.js (8)
- kotlin (2)
- last.fm api (2)
- linq (1)
- mac (2)
- macos (1)
- mathematica (1)
- msbuild (3)
- mvc core (3)
- nancy (2)
- native (1)
- net (141)
- net 5 (3)
- net 6 (5)
- net 7 (7)
- net 8 (3)
- net 9 (1)
- net core (49)
- net sdk (2)
- ninject (2)
- odata (4)
- oidc (2)
- omnisharp (13)
- openai (9)
- osx (2)
- owin (5)
- php (1)
- q-sharp (34)
- qir (3)
- quantum computing (37)
- roslyn (30)
- rust (4)
- scriptcs (11)
- scripting (9)
- security (6)
- servicestack (2)
- signalr (7)
- swift (7)
- testing (5)
- twitter boostrap (1)
- typescript (1)
- visual studio (4)
- visual studio code (11)
- wasi (3)
- wasm (3)
- windows phone 7 (1)
- wordpress (1)
- wpf (2)