Global route prefixes with attribute routing in ASP.NET Web API
As you may have learnt from some of the older posts, I am a big fan, and a big proponent of attribute routing in ASP.NET Web API.
One of the things that is missing out of the box in Web API’s implementation of attribute routing, is the ability to define global prefixes (i.e. a global “api” that would be prepended to every route) - that would allow you to avoid repeating the same part of the URL over and over again on different resources. However, using the available extensibility points, you can provide that functionality yourself.
Let’s have a look.
Using Roslyn and unit tests to enforce coding guidelines and more
Last year, during a few of my Roslyn talks, I was presenting a cool idea of leveraging Roslyn in unit tests to enforce a certain style in code, and in general inspect the consistency of the code in various ways.
It’s a really powerful concept, and something I wanted to blog about, but of course forgot - until I was reminded of that yesterday on Twitter.
Let’s have a look.
Running a C# REPL in a DNX application with scriptcs
One of the cool things that scriptcs allows you to do, is that you can embed it into your application and allow execution of C# scripts. There are even some great resources on that out there, like this awesome post by Mads.
The same applies to the REPL functionality - you don’t have to use scriptcs.exe to access the REPL - you can use the scriptcs Nuget packages to create a REPL inside your app.
And because there aren’t that many resources (if any) on how to host a scriptcs REPL, today I wanted to show you just that. But for a more interesting twist, we’ll do that inside a DNX application.
There are many reasons why DNX is awesome, and why you’d want to use it, but especially because, through the project.json project system, it has a much improved way of referencing and loading dependencies and Nuget packages - and we can leverage that mechanism to feed assemblies into our REPL.
Hacking DNX to run C# scripts
Because of my considerable community involvement in promoting C# scripting (i.e. here or here), I thought the other day, why not attempt to run C# scripts using DNX?
While out of the box, DNX only compiles proper, traditional C# only, thanks to the compilation hooks it exposes, it is possible to intercept the compilation object prior to it being actually emitted, which allows you to do just about anything - including run C# scripts.
Let’s explore more.
Disposing resources at the end of Web API request
Sometimes you have resources in your code that are implementing IDisposable, and that you’d like them to be disposed only at the end of the HTTP request. I have seen a solution to this problem rolled out by hand in a few code bases in the past - but in fact this feature is already built into Web API, which I don’t think a lot of people are aware of.
Let’s have a quick look.
ViewComponents in ASP.NET 5 and ASP.NET MVC 6
Let’s have a quick look at another new feature in ASP.NET MVC 6, and that is the ViewComponent feature. View components are intended to be replacements to ChildActions and, to some extent, of partial views.
Traditionally in ASP.NET MVC (and in general in the textbook MVC pattern), you had to compose the entire model in the controller and pass it along to the view, which simply rendered the entire page based on the data from the model. The consequence of this is that the view does not need to explicitly ask for any data - as its sole purpose is to just act upon the model it received.
While this sounds very nice in theory, it has traditionally posed a number of practical difficulties. There are a number of reusable components on pretty much every website - think a menu, a shopping cart, lists of all kinds, breadcrumbs, metadata and so on - so things that appear on multiple pages.
Let’s have a look at how this is solved in MVC 6.
Migrating from Web API 2 to MVC 6 at NDC Oslo
Action filters, service filters and type filters in ASP.NET 5 and MVC 6
Today, let’s have a look at he area of filters in ASP.NET MVC 6 - because it actually contains quite a few interesting changes compared to classic MVC and Web API filter pipelines.
Let’s leave the specialized filters (error filters, authorization filters) on a side for now, and focus instead on the functional, aspect oriented, filters. Aside from the good old action filters, known from both MVC and from Web API, there are two new types of filters (or rather filter factories, but we’ll get there) that you can use - ServiceFilters and TypeFilters.
Integration testing ASP.NET 5 and ASP.NET MVC 6 applications
The other day I ran into a post by Alex Zeitler, who blogged about integration testing of ASP.NET MVC 6 controllers. Alex has done some great work for the Web API community in the past and I always enjoy his posts.
In this case, Alex suggested using self hosting for that, so spinning up a server and hitting it over HTTP and then shutting down, as part of each test case. Some people have done that in the past with Web API too, but is not an approach I agree with when doing integration testing. If you follow this blog you might have seen my post about testing OWIN apps and Web API apps in memory already.
My main issue with the self-host approach, is that you and up testing the underlying operating system networking stack, the so-called “wire” which is not necessarily something you want to test - given that it will be different anyway in production (especially if you intend to run on IIS). On the other hand, you want to be able to run end-to-end tests quickly anywhere - developer’s machine, integration server or any other place that it might be necessary, and doing it entirely in memory is a great approach.
ASP.NET MVC 6 formatters – XML and browser requests
A while ago I wrote a post about formatters in MVC 6.
Since then, there have been some changes regarding XML handling and an interesting feature that was added recently that was not part of that post, so I think it warranties a follow up. XML formatter is now removed by default. On top of that, MVC 6 will attempt to sniff out whether your request is originating from a browser’s address bar and adjust content negotiation accordingly.
About
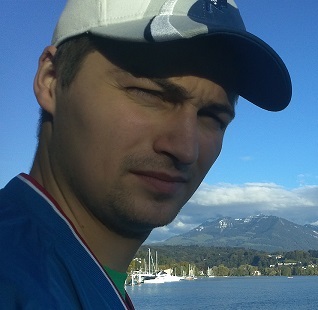
Hi! I'm Filip W., a cloud architect from Zürich 🇨🇭. I like Toronto Maple Leafs 🇨🇦, Rancid and quantum computing. Oh, and I love the Lowlands 🏴.
You can find me on Github and on Mastodon.
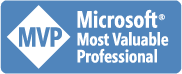
Recent Posts
- 2024/04/19, Tool Calling with Azure OpenAI - Part 2: Using the tools directly via the SDK
- 2024/04/04, Tool Calling with Azure OpenAI - Part 1: The Basics
- 2024/03/08, Combining Azure OpenAI with Azure AI Speech
- 2024/02/23, Using your own data with GPT models in Azure OpenAI - Part 4: Adding vector search
- 2024/01/31, Running Swift Package tests in Azure DevOps
Categories
- ai (9)
- ai search (4)
- apache cordova (1)
- asp.net 5 (17)
- asp.net core (45)
- asp.net mvc (35)
- asp.net mvc 6 (7)
- asp.net vnext (6)
- asp.net web api (96)
- astronomy (1)
- azure (19)
- azure service bus (1)
- azure-devops (1)
- benchmark dotnet (1)
- bing maps (1)
- blazor (1)
- c plus (2)
- c-sharp (154)
- cryptography (3)
- csharp (2)
- csharp 10 (2)
- dnx (3)
- dotnet-cli (2)
- dotnet-script (11)
- duende (2)
- editorconfig (1)
- entity framework (2)
- espn api (2)
- events (1)
- ffi (3)
- fsharp (1)
- git (1)
- glimpse (1)
- html5 (4)
- identity server (2)
- iis (2)
- il (1)
- intro to qc (19)
- ios (2)
- javascript (9)
- jquery (4)
- jquery mobile metro (1)
- katana (2)
- kindle (1)
- knockout.js (8)
- last.fm api (2)
- linq (1)
- mac (2)
- macos (1)
- mathematica (1)
- msbuild (3)
- mvc core (3)
- nancy (2)
- native (1)
- net (141)
- net 5 (3)
- net 6 (5)
- net 7 (7)
- net 8 (3)
- net core (49)
- net sdk (2)
- ninject (2)
- odata (4)
- oidc (2)
- omnisharp (13)
- openai (9)
- osx (2)
- owin (5)
- php (1)
- q-sharp (33)
- qir (2)
- quantum computing (36)
- roslyn (30)
- rust (2)
- scriptcs (11)
- scripting (9)
- security (6)
- servicestack (2)
- signalr (7)
- swift (4)
- testing (5)
- twitter boostrap (1)
- typescript (1)
- visual studio (4)
- visual studio code (11)
- wasi (3)
- wasm (3)
- windows phone 7 (1)
- wordpress (1)
- wpf (2)